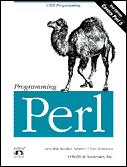
|
|
CGI Programming with Perl
Lesson 1: Getting Started with Perl
| Type | Example |
$ | Scalar | $name = "Gary Patterson"; |
@ | Array | @colors = (red, green, blue); |
% | Hash | %mfr("747") = "Boeing"; |
& | Subroutine | &display("Please wait..."); |
* | Typeglob | *filehandle; |
Wrong | Right |
#! /usr/bin/local/perl
$input = <STDIN>;
if ($input ne "") {
print($input);
} else {
print("no input");
}
|
#! /usr/bin/local/perl
$input = <STDIN>;
if ($input ne "\n") {
print($input);
} else {
print("no input");
}
|
Perl's <STDIN> from the keyboard appends a newline to the input. When testing for a keyboard empty line, use ($x eq "\n") instead of ($x eq ""). To avoid this, use the chomp($input); code. |
#! /usr/bin/local/perl
@c=('red','green','blue');
for ($i=0; $i<@c; $i++) {
print('$c[$i]\n');
}
|
#! /usr/bin/local/perl
@c=('red','green','blue');
for ($i=0; $i<@c; $i++) {
print("$c[$i]\n");
}
|
Notice the double quotes in the print() command parameter. It forces the variables to be interpreted by reference instead of literals. |
Exercises
1 |
perl -e "print(\"John Doe\n\")"; -e "print(\"123 Main St\n\")"; -e "print(\"Anytown, MA 12345\")";
perl -e "print qq(John Doe\n123 Main Street\nAnytown, USA 12345\n)"
| |
2 |
print("Enter your Name: ");
$name = <STDIN>;
chomp($name);
if ($name eq "") {
$name = "No name given";
}
print("Enter your Address: ");
$addr = <STDIN>;
chomp($addr);
if ($addr eq "") {
$addr = "No address given";
}
print("Enter your City, State and Zip Code: ");
$CSZ = <STDIN>;
chomp($CSZ);
if ($CSZ eq "") {
$CSZ = "No City, State, and Zip Code given";
}
print("$name\n$addr\n$CSZ\n");
| |
3 |
$last = <STDIN>;
chomp($last);
$first = <STDIN>;
chomp($first);
$addr = <STDIN>;
chomp($addr);
$city = <STDIN>;
chomp($city);
$state = <STDIN>;
chomp($state);
$zip = <STDIN>;
chomp($zip);
print("$first $last\n");
print("$addr\n");
print("$city, $state $zip\n");
perl sourcefile.pl <datafile
| |
4 |
$line = <STDIN>;
while ($line ne "") {
print($line);
$line = <STDIN>;
}
while ($line = <STDIN>) {
print $line;
}
perl sourcefile.pl <inputfile >outputfile
| |
fatpat Software · PO Box 1785 · Charlottesville, VA 22902 · (804) 977-1652
|